How do I make a simple text chat website?
3 min read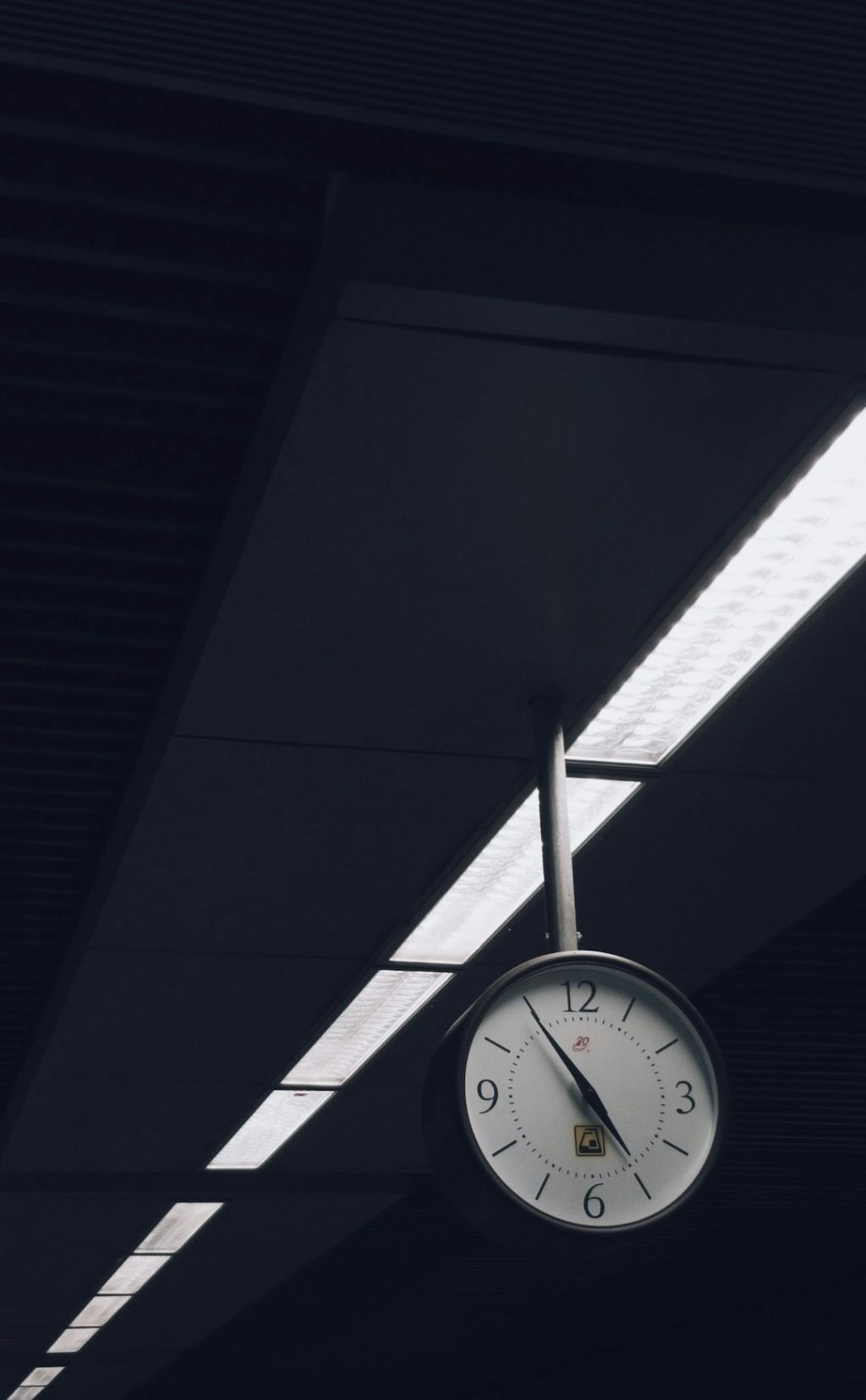
Creating a simple text chat website is a fun and educational project for aspiring web developers. Not only does it introduce you to core web technologies like HTML, CSS, and JavaScript, but it can also teach you basic client-server communication using tools like Socket.IO and Node.js. In this article, you’ll learn how to build a basic chat application you can run locally or host online. Whether you’re a hobbyist or just getting started in web development, this guide offers an exciting gateway into real-time web communication.
What You’ll Need
- A code editor (e.g., VS Code)
- Node.js and npm installed
- Basic understanding of HTML, CSS, and JavaScript
Once you’re set up, follow these main steps to build your chat website:
1. Set Up the Project Folder
Create a new folder for your project, and inside it, add the following files:
- index.html – The HTML page for your chat UI
- style.css – To style your chat interface
- script.js – Handles client-side chat logic
- server.js – A Node.js server script
2. Build the Chat Interface
Start with index.html and create a simple UI where users can type and send messages. Here’s a basic example:
<!DOCTYPE html> <html> <head> <title>Simple Chat</title> <link rel="stylesheet" href="style.css"> </head> <body> <div id="chat-box"></div> <form id="chat-form"> <input id="message" autocomplete="off" placeholder="Type a message..." /> <button>Send</button> </form> <script src="/socket.io/socket.io.js"></script> <script src="script.js"></script> </body> </html>
The chat box will display messages, and the form at the bottom will accept user input.
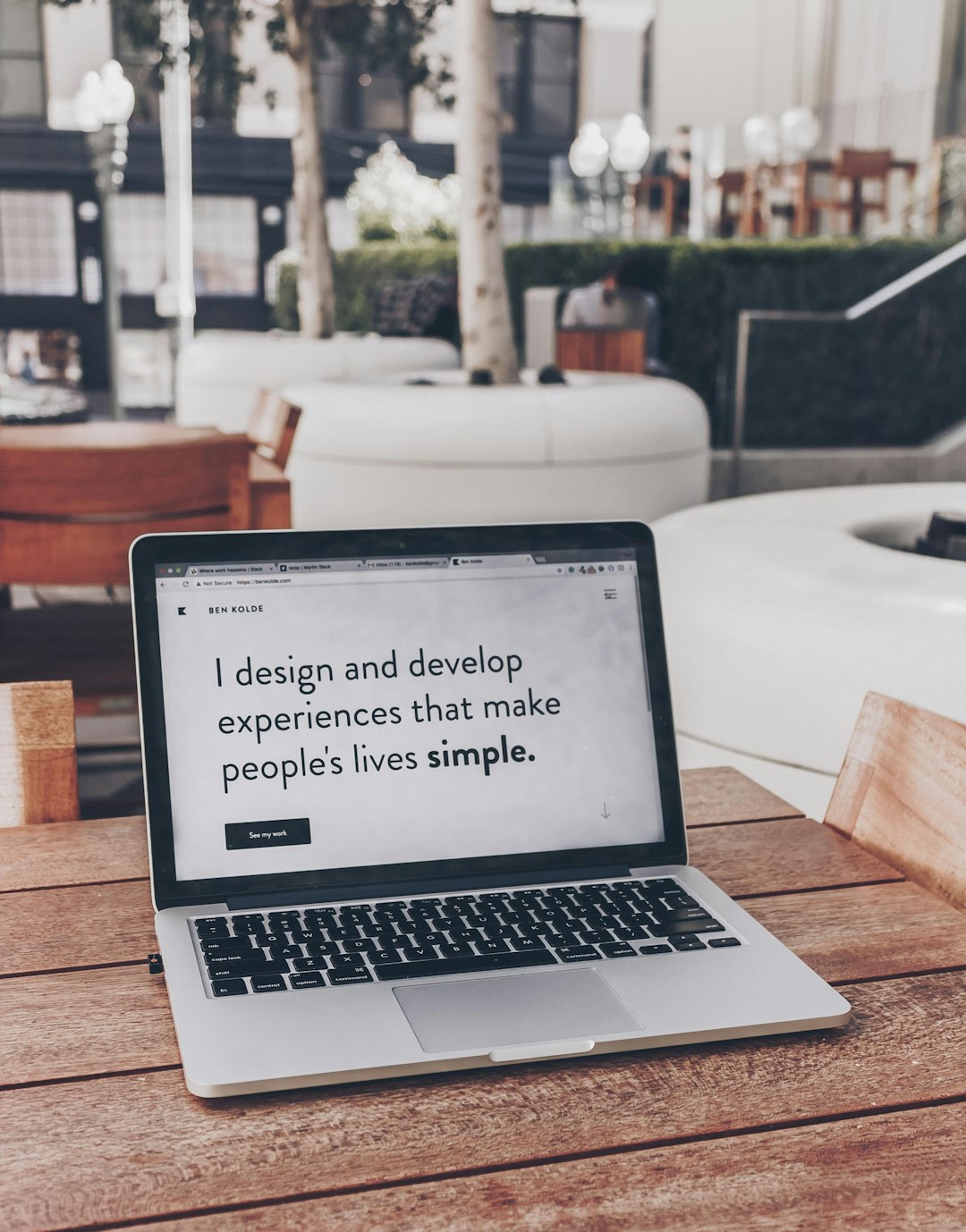
3. Add Some Style
Open style.css to style your interface. While you can customize it however you like, here’s a minimal example:
body { font-family: Arial, sans-serif; margin: 0; padding: 20px; } #chat-box { height: 300px; overflow-y: scroll; border: 1px solid #ccc; padding: 10px; margin-bottom: 10px; } #chat-form { display: flex; } #message { flex: 1; padding: 10px; }
Now your interface has a functional layout for real-time interaction.
4. Set Up the Server
In server.js, you’ll create a basic Node server using Express and Socket.IO for real-time communication:
const express = require('express'); const app = express(); const http = require('http').createServer(app); const io = require('socket.io')(http); app.use(express.static(__dirname)); io.on('connection', (socket) => { console.log('A user connected'); socket.on('chat message', (msg) => { io.emit('chat message', msg); }); socket.on('disconnect', () => { console.log('A user disconnected'); }); }); http.listen(3000, () => { console.log('Server running on http://localhost:3000'); });
This allows multiple clients to connect and exchange messages through the server.
5. Connect the Frontend and Backend
Finally, in your script.js, you’ll add the code to send and receive messages via the socket:
const socket = io(); const form = document.getElementById('chat-form'); const input = document.getElementById('message'); const chatBox = document.getElementById('chat-box'); form.addEventListener('submit', function(e) { e.preventDefault(); if (input.value) { socket.emit('chat message', input.value); input.value = ''; } }); socket.on('chat message', function(msg) { const item = document.createElement('div'); item.textContent = msg; chatBox.appendChild(item); chatBox.scrollTop = chatBox.scrollHeight; });
With this setup, sending a message updates all connected users in real time.
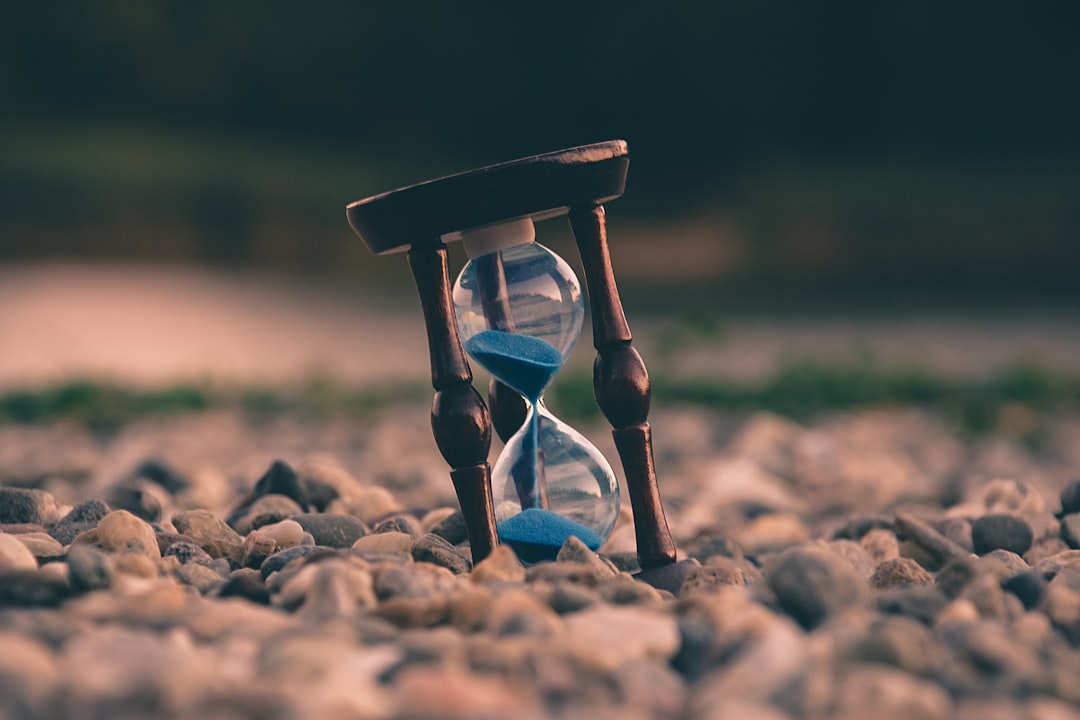
6. Run Your Chat App
Open your terminal and navigate to the folder containing your files. Run the following command:
node server.js
Visit http://localhost:3000 in your browser to see your chat website in action. Open multiple tabs or browsers to simulate multiple users chatting live!
Final Thoughts
Building a simple chat application from scratch is a practical way to learn full-stack web development. Once you’re comfortable, you can expand the app with features like usernames, rooms, authentication, and persistence via databases. You could even deploy it using services like Heroku or Vercel.
Whether you’re just starting out or practicing your skills, this project offers a meaningful and interactive way to understand real-time web applications.